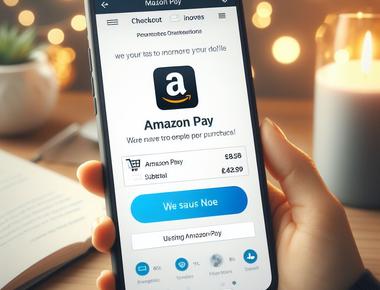
這是我第二次接手 Amaozn pay,上次是因為剛加入公司時,幫忙處理Amazon pay 一直瘋狂掉單,這次重新接 Amazon pay 是為了德國的電商網站,藉由此次重新整理了一下界接的技巧。
Amazon pay checkout 其實就很像 payapal express 的 checkout
ssh-keygen -t rsa -b 2048 -m PKCS8 -f privateKey.pem ssh-keygen -f privateKey.pem -e -m PKCS8 > publicKey.pub